Testing landing pages
Testing landing pages will help you hypothesize, see where users click more often, as well as edit your landing pages depending on their behavior.
Tracking exit points
If a landing page have multiple exit points to an offer, try to track them.
To do so, add the following parameter to your Click URL on LPs: &place=exit_point_name
.
As a result, you will get the following type of report in the campaign’s statistics:
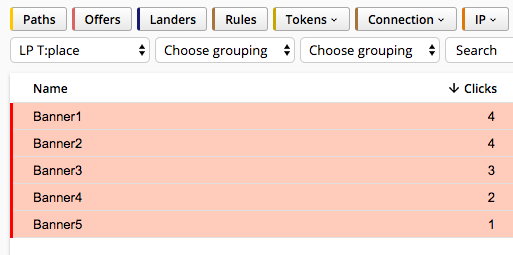
Multivariate testing
Sometimes an ordinary A/B testing is not enough for effective landing page analyze. In this case multivariate testing (MVT) can help. Its main advantage is to combine and test different versions of images, buttons, texts, and other elements inside one page, and the statistics in the tracker will help you find the best variant.
In order to see the stats you have to use LP Tokens or Events with our randomization code.
A landing page should have the .php and contain the following code at the very beginning (before <!DOCTYPE html>):
<?php
function getRandomElement( $arr ){
$keys = array_keys($arr);
$len = count( $keys );
$rand = mt_rand( 0, $len-1 );
$index = $keys[$rand];
return [$index, $arr[ $index ]];
}
function makeUpdateTokenURL( $TRACKER_URL, $indexes ){
$s = $TRACKER_URL.'/click.php?lp=data_upd&';
foreach ($indexes as $tokenName => $tokenValue) {
$s .= "{$tokenName}={$indexes[$tokenName]}&";
}
$s = rtrim($s, "&");
return $s;
}
function getChosen( $blockSet, $randomOptions ){
$indexes = array();
$result = array();
foreach ($blockSet as $blockName=>$values) {
$indexes[$blockName] = [];
$result[$blockName] = [];
if ( !isset($randomOptions[$blockName]) || $randomOptions[$blockName]==="random" ){
list($index, $element) = getRandomElement( $values );
} else {
$index = $randomOptions[$blockName];
$element = $values[$index];
}
$indexes[$blockName] = $index;
$result[$blockName] = $element;
}
return [ $indexes, $result ];
}
Then you need to add the main part with your content options. For example, you want to test 3 different headers, 3 different pictures and 3 different font colors:
// Specify your campaign domain
$TRACKER_DOMAIN = 'https://your-campaign-domain.com';
// Specify name of LP token for tracker, its value and code content of the main page
$bricks = [
// First header token and its options
'header'=> [
"1" => '<h1>Test 1</h1>',
"2" => '<h1>Test 2</h1>',
"3" => '<h1>Test 3</h1>'
],
// Second wallpaper token and its options
'wallpaper'=> [
"1" => '<img src="images/wallpaper-1.png">',
"2" => '<img src="images/wallpaper-2.png">',
"3" => '<img src="images/wallpaper-3.png">'
],
// Third font-color token and its options
'font-color'=> [
"Green" => '#41f449',
"Yellow" => '#f4f141',
"Red" => '#f44242'
]
];
Now you need to choose whether you substitute elements randomly or fix a specific variant.
$randomOptions = [
// Only the first header
"header" => 1,
// All the options
"wallpaper" => "random",
"font-color" => "random",
];
Then you need to add another block (nothing to change here):
list( $indexes, $result ) = getChosen( $bricks, $randomOptions );
$updateTokensURL = makeUpdateTokenURL( $TRACKER_DOMAIN, $indexes );
$updateLPTokensScript = <<<EOT
<script type="text/javascript">
var o = document.createElement("img");
o.src="{$updateTokensURL}";
</script>
EOT;
?>
In the end, add the main code of your page with all the relevant content:
<!DOCTYPE html>
<html>
<head>
<title>Test!</title>
<!-- Passing data to the tracker -->
<?php echo $updateLPTokensScript; ?>
</head>
<body>
<h1>
<!-- Specify the first element -->
<?= $result["header"]; ?>
</h1>
<!-- Specify the second element -->
<?= $result["wallpaper"]; ?>
<!-- Specify the third font-color token -->
<a style="color:<?= $result["font-color"]; ?>;font-size:300%;text-decoration-line:none" href="https://tracker.com/click.php?lp=1"> Link to the offer </a>
</body>
</html>
Finally you will get a .php file like this:
-
Final code
-
<?php function getRandomElement( $arr ){ $keys = array_keys($arr); $len = count( $keys ); $rand = mt_rand( 0, $len-1 ); $index = $keys[$rand]; return [$index, $arr[ $index ]]; } function makeUpdateTokenURL( $TRACKER_URL, $indexes ){ $s = $TRACKER_URL.'/click.php?lp=data_upd&'; foreach ($indexes as $tokenName => $tokenValue) { $s .= "{$tokenName}={$indexes[$tokenName]}&"; } $s = rtrim($s, "&"); return $s; } function getChosen( $blockSet, $randomOptions ){ $indexes = array(); $result = array(); foreach ($blockSet as $blockName=>$values) { $indexes[$blockName] = []; $result[$blockName] = []; if ( !isset($randomOptions[$blockName]) || $randomOptions[$blockName]==="random" ){ list($index, $element) = getRandomElement( $values ); } else { $index = $randomOptions[$blockName]; $element = $values[$index]; } $indexes[$blockName] = $index; $result[$blockName] = $element; } return [ $indexes, $result ]; } // Your campaign domain $TRACKER_DOMAIN = 'https://your-campaign-domain.com'; // Specify the name of LP token for the tracker, its value, and code content of the main page $bricks = [ // The first header token and its options 'header'=> [ "1" => '<h1>Test 1</h1>', "2" => '<h1>Test 2</h1>', "3" => '<h1>Test 3</h1>' ], // The second wallpaper token and its options 'wallpaper'=> [ "1" => '<img src="images/wallpaper-1.png">', "2" => '<img src="images/wallpaper-2.png">', "3" => '<img src="images/wallpaper-3.png">' ], // The third font-color token and its options 'font-color'=> [ "Green" => '#41f449', "Yellow" => '#f4f141', "Red" => '#f44242' ] ]; $randomOptions = [ // Only the first header "header" => 1, // All options "wallpaper" => "random", "font-color" => "random" ]; list( $indexes, $result ) = getChosen( $bricks, $randomOptions ); $updateTokensURL = makeUpdateTokenURL( $TRACKER_DOMAIN, $indexes ); $updateLPTokensScript = <<<EOT <script type="text/javascript"> var o = document.createElement("img"); o.src="{$updateTokensURL}"; </script> EOT; ?> <!DOCTYPE html> <html> <head> <title>Test!</title> <!-- Sending data to the tracker --> <?php echo $updateLPTokensScript; ?> </head> <body> <h1> <!-- Specify the first element --> <?= $result["header"]; ?> </h1> <!-- Specify the second element --> <?= $result["wallpaper"]; ?> <!-- Specify the third element --> <a style="color:<?= $result["font-color"]; ?>;font-size:300%;text-decoration-line:none" href="https://tracker.com/click.php?lp=1"> Offer link </a> </body> </html>
-
If you want to have a landing page via a direct link, use it with Click API. In this case your landing page code will look like this:
-
Final code with Click API
-
<?php function getRandomElement( $arr ){ $keys = array_keys($arr); $len = count( $keys ); $rand = mt_rand( 0, $len-1 ); $index = $keys[$rand]; return [$index, $arr[ $index ]]; } function makeUpdateTokenURL( $TRACKER_URL, $indexes ){ $s = $TRACKER_URL.'/click.php?lp=data_upd&'; foreach ($indexes as $tokenName => $tokenValue) { $s .= "{$tokenName}={$indexes[$tokenName]}&"; } $s = rtrim($s, "&"); return $s; } function getChosen( $blockSet, $randomOptions ){ $indexes = array(); $result = array(); foreach ($blockSet as $blockName=>$values) { $indexes[$blockName] = []; $result[$blockName] = []; if ( !isset($randomOptions[$blockName]) || $randomOptions[$blockName]==="random" ){ list($index, $element) = getRandomElement( $values ); } else { $index = $randomOptions[$blockName]; $element = $values[$index]; } $indexes[$blockName] = $index; $result[$blockName] = $element; } return [ $indexes, $result ]; } // Your tracker's domain $TRACKER_DOMAIN = 'https://tracker.com'; // Adding Click API include 'binom_click_api.php'; // Specify the name of LP token for the tracker, its value, and code content of the main page $bricks = [ // The first header token and its options 'header'=> [ "1" => '<h1>Test 1</h1>', "2" => '<h1>Test 2</h1>', "3" => '<h1>Test 3</h1>' ], // The second wallpaper token and its options 'wallpaper'=> [ "1" => '<img src="images/wallpaper-1.png">', "2" => '<img src="images/wallpaper-2.png">', "3" => '<img src="images/wallpaper-3.png">' ], // The third font-color token and its options 'font-color'=> [ "Green" => '#41f449', "Yellow" => '#f4f141', "Red" => '#f44242' ] ]; $randomOptions = [ // Only the first header "header" => 1, // All options "wallpaper" => "random", "font-color" => "random" ]; list( $indexes, $result ) = getChosen( $bricks, $randomOptions ); // Sending data to the tracker $updateTokensURL = makeUpdateTokenURL( $TRACKER_DOMAIN, $indexes ); $send_request = file_get_contents($updateTokensURL.'&uclick='.$getClick->DataClick['uclick']); ?> <!DOCTYPE html> <html> <head> <title>Test!</title> </head> <body> <h1> <!-- Specify the first element --> <?= $result["header"]; ?> </h1> <!-- Specify the second element --> <?= $result["wallpaper"]; ?> <!-- Specify the font-color token with font colors and sizes --> <a style="color:<?= $result["font-color"]; ?>;font-size:300%;text-decoration-line:none" href="https://tracker.com/click.php?lp=1"> Offer link </a> </body> </html>
-
Keep in mind that you need to have the binom_click_api.php file in the same folder with your landing page. Make sure to specify both your campaign link and API key in the file.